Flutter: google signin without firebase
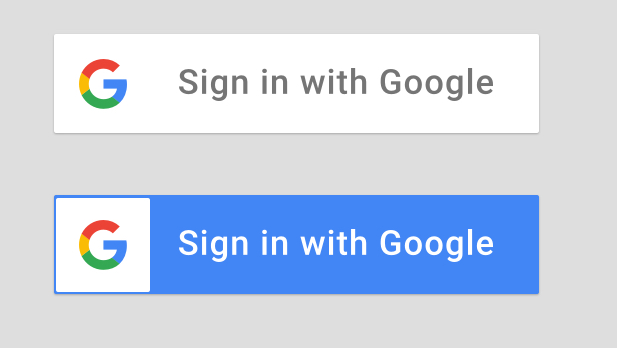
I'm working on integrating nakama as backend for my flutter-projects and I do want to use google_sign_in for authentication on android. In order to use nakama's authenticateGoogle(...) you need to bypass a clientId-token, but how to get this?
Out of the box this value seems to be 'null' on the plugin's response-object. Crawling the internet all(?) results I found were using firebase to some extend (in the end just to create this magical google-services.json ). But since nakama is my backend of choice, it felt(!) wrong to create a firebase-project along nakama π
Create google project for signing in:
That works surprisingly easy(whoever already opened google's developer-console know what I mean π):
- go to https://developers.google.com/identity/sign-in/android/start-integrating
- Press the 'Configure a project'-Button

- (I guess it might be possible that you need to signup for google cloud. I didn't need this, because I already did before)
- Select an exisiting project or create a new one
- Fill in product-name
- Where are you calling from => Android
- Fill in package-name: You find this in [FlutterProject]/android/app/build.gradle (search for 'applicationId') e.g. org.mysample.thetest
- In order to get the SHA-1 signing certificate, open a terminal in your [FlutterApp]/android-folder and type:
./gradlew signingReport
This will spit out something like this(no worry I invalidated following sample keys):
...
----------
Variant: debugUnitTest
Config: debug
Store: /home/bla/.android/debug.keystore
Alias: AndroidDebugKey
MD5: B2:FF:D8:FF:AB:FF:04:35:FF:50:20:0E:C5:8F:D8:FF
SHA1: 2F:79:23:FF:11:FF:F5:FF:4F:F:AA:95:96:BA:C0:B2:45:A0:B7:83
SHA-256: E0:38:29:FF:FF:DA:FF:66:D1:4D:E1:FF:3C:06:D0:1B:24:6F:F6:98:31:46:EC:C2:D5:91:8A:88:8E:79:FF:FF
Valid until: Sunday, 11 September 2050
----------
...
- copy & paste the SHA1-Fingerprint to the web-interface
- Hit the 'create'-button
- Press the Button 'DOWNLOAD CLIENT CONFIGURATION' and save it somewhere you'll find it again
- Create a file 'signin.xml' (name it, as you want) in the folder:[FlutterApp]/android/app/src/main/res and paste following and replace [client-id] with the client id you just created :
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="default_web_client_id">[client-id]</string>
</resources>
- (If you already closed the browser, look in the file you saved before or go to the google-console and search the OAuth-Client-Data )
- Now you are ready to receive not only the user profile but also the client-id-token for your backend.
- Just for info: You likely have to repeat this process with your release-keys before going live with your app
You can query the client-id-token with something like that:
GoogleSignIn _googleSignIn = GoogleSignIn(
scopes: ['profile', 'email'],
);
Future<void> _handleSignIn() async {
try {
var account = await _googleSignIn.signIn();
var googleKey = await account.authentication;
googleSignInidToken = googleKey.idToken;
print("AccessToken: ${googleKey.accessToken}");
print("idToken: ${googleKey.idToken}");
print("serverAuthCode: ${googleKey.serverAuthCode}");
} catch (error) {
print(error);
}
}
PS: I'm actually not sure why I had the stronge urge to avoid firebase. In the end it is just an additional set of google-apis.π